Introduction

The proliferation of chatbots in the technology industry has been a burgeoning topic of interest lately, and it’s easy to see why. These conversational agents offer a tremendous value proposition, enabling businesses to improve customer service, drive customer engagement, and boost product sales. In this article, we’ll delve into the nitty-gritty details of how to hard-code a chatbot using Python programming language. Once you’ve succeeded in the coding process, we’ll furnish you with comprehensive instructions on how to go about deploying your newly-created chatbot.
What Is A Chatbot?
The rudimentary construct of a chatbot is that of a computer program that possesses the ability to simulate human conversation, effectively serving as an conversational partner Primarily utilized to dispense customer service or technical support, chatbots can also be effectively deployed for marketing or even sales. While there are several distinct methodologies to code a chatbot, the current exposition shall be focused specifically on elucidating the intricacies of hard-coding a chatbot using Python, i.e to leverage the highly dynamic and adaptable Python programming language.
Why Create A Chatbot Using Python?
Python is an absolutely top-notch programming language when it comes to crafting chatbots, given its innumerable libraries that enable the creation of a multitude of Natural Language Processing (NLP) models. For the uninitiated, NLP refers to the realm of computer science that revolves around imparting the ability to computers to comprehend human language. It is a fundamental constituent of designing chatbots that can convincingly replicate the tenor and texture of human conversation.
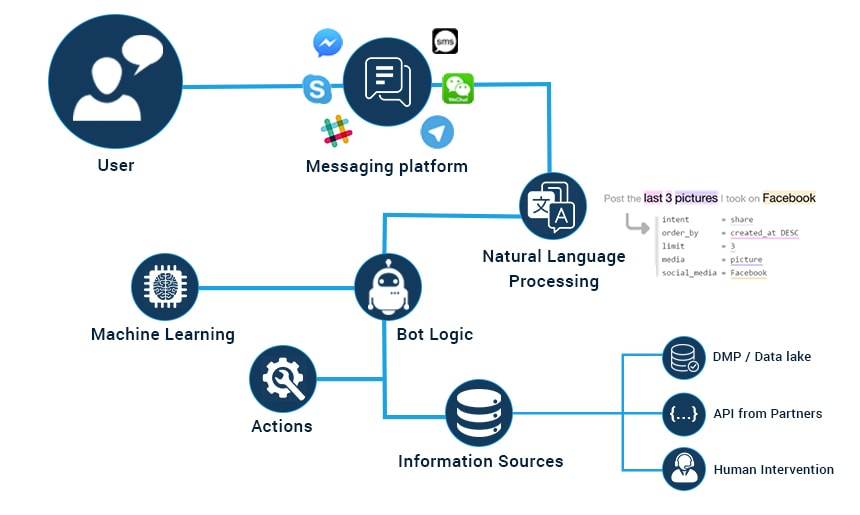
The Python ecosystem boasts of an exhaustive array of NLP libraries, each catering to a unique set of requirements. However, in this article, our focus shall rest on the NLTK library. The Natural Language Toolkit (NLTK) is a highly sought-after and widely adopted NLP library that avails us the entire gamut of resources necessary to fabricate our chatbot with consummate ease.
Creating The Chatbot Using Python
To embark upon the journey of constructing our very own chatbot via the utilization of Python programming language, the foremost step is to procure the quintessential Natural Language Toolkit (NLTK) library. An action that can be readily executed through the implementation of the ensuing command in our terminal:
pip install nltk
Once we have successfully completed the installation of the NLTK library, we can seamlessly import it into our Python file by means of the ensuing code:
import nltk
nltk.download(‘punkt’)
The aforementioned code snippet will serve as the impetus for importing the NLTK library into our programming framework, simultaneously catalyzing the download of the Punkt tokenizer. The Punkt tokenizer, being a critical component of Natural Language Processing, comes into play when dissecting human language into smaller segments known as tokens, thereby enabling the processing and comprehension of varied components of speech constituting a human conversation.
With the preceding step behind us, the subsequent course of action necessitates the development of a bespoke function that enables the tokenization of a given sentence via the Punkt tokenizer. This can be achieved through the deployment of the ensuing code snippet:
def tokenize(sentence):
tokens = nltk.word_tokenize(sentence)
return tokens
Having successfully fashioned our customized tokenize function, we can now proceed towards the development of another function that takes as input a sentence, subsequently tokenizes it, and then classifies the diverse parts of speech for each word in the sentence. This can be executed via the utilization of the ensuing code snippet:
def pos_tagging(sentence):
tokens = tokenize(sentence)
tagged_tokens = nltk.pos_tag(tokens)
return tagged_tokens
Now that we have reached an advanced stage of the chatbot creation process, it’s time to devise a function that incorporates the pos_tagging function we developed earlier, enabling us to tokenize a given sentence, classify its different parts of speech, and subsequently identify the subject of the sentence. This can be accomplished by implementing the ensuing code snippet:
def find_subject(sentence):
tagged_tokens = pos_tagging(sentence)
for i in range(0, len(tagged_tokens)):
if (tagged_tokens][i][1] == ‘NNP’) or (tagged_tokens][i][1] == ‘NN’):
return tagged_tokens][i][0]
And finally as we have our highly specialized find_subject function in place, we can effortlessly forge ahead to develop yet another function that’s capable of taking as input a sentence, tokenizing it, classifying the parts of speech, identifying the subject, and then generating a prompt response. The following code snippet elucidates the inner workings of this function:
def generate_response(sentence):
subject = find_subject(sentence)
response = “I’m sorry, I don’t know anything about ” + subject + “.”
return response
If we run our code with the sentence “What is your favorite color?”, we should get the ensuing output:
I’m sorry, I don’t know anything about color.
As is evidently manifested by the outcome of this experiment, our chatbot has been equipped with the inherent capabilities to comprehend the subject of the sentence and correspondingly generate a response, paving the way for seamless communication between the user and the chatbot.
Chatbot Deployment
When it comes to deploying a chatbot, the process can be quite multifaceted, with several potential approaches at our disposal. However, in this particular discussion, we will be honing in on the deployment capabilities of the ubiquitous Flask framework.
As a web framework written in Python, Flask affords developers considerable flexibility in crafting dynamic web applications. Its rich feature set makes it an ideal candidate for chatbot deployment, providing ample opportunity for customization and scalability.
The framework’s functionalities allow for effortless deployment of chatbots as web applications.
To initialize, the first step is to establish Flask on our system by executing the following command in the terminal:
pip install flask
Following successful installation, the subsequent step is to create a Python file named “app.py,” where the Flask framework is imported utilizing the subsequent code snippet:
from flask import Flask, request
app = Flask(name)
By incorporating Flask, an object of the Flask application is produced, granting the ability to design the chatbot’s behavior.
In our quest to share our chatbot with the wider public, we enlist the services of the formidable Flask framework, a dynamic and powerful instrument in the Python developer’s arsenal. With a well-defined set of instructions, we can effortlessly make our chatbot available to the world.
The first step on this journey involves installing the Flask framework, a straightforward process that can be executed via the command prompt with a simple yet powerful command:
pip install flask
After the installation of the Flask, generate a file named app.py, to house the chabot’s logic. Well, now, we can import the Flask framework using the following code:
from flask import Flask, request
app = Flask(name)
Now with that done, we can create a Flask application object that can handle incoming web requests with skill and readiness.
Moving on, to establish a route for our chatbot, we utilize the ensuing code snippet:
@app.route(‘/chatbot’, methods=[‘GET’, ‘POST’])
def chatbot():
return “Greetings, human!”
This code generates a route labeled /chatbot, where the chatbot is accessed. Upon access, the route will reply with the phrase “Greetings, human!”
With the Flask application set up, we can proceed to run it, enabling access to our chatbot. Running the subsequent command in the terminal will enable us to do this:
python app.py
By visiting http://localhost:5000/chatbot in our web browser, we are then presented with the following outcome:
Greetings, human!
The preceding outcome verifies that our chatbot is now accessible at http://localhost:5000/chatbot, and can be interacted with using a web browser.
In conclusion, by harnessing the power of the Flask framework, we can expeditiously deploy our chatbot to a web application. The preceding procedure necessitated only a few lines of code, effectively making our chatbot globally accessible to anyone with an internet connection.
Chatbot Testing
Upon completion of the chatbot creation process using Python, the world is your oyster. You can leverage your newly-created chatbot to dispense customer service, enthrall customers, or even effectuate sales. However, prior to the actual chatbot deployment, it is prudent to perform a rigorous chatbot testing regimen. One such invaluable service for chatbot testing is the much-touted Botium.ai.
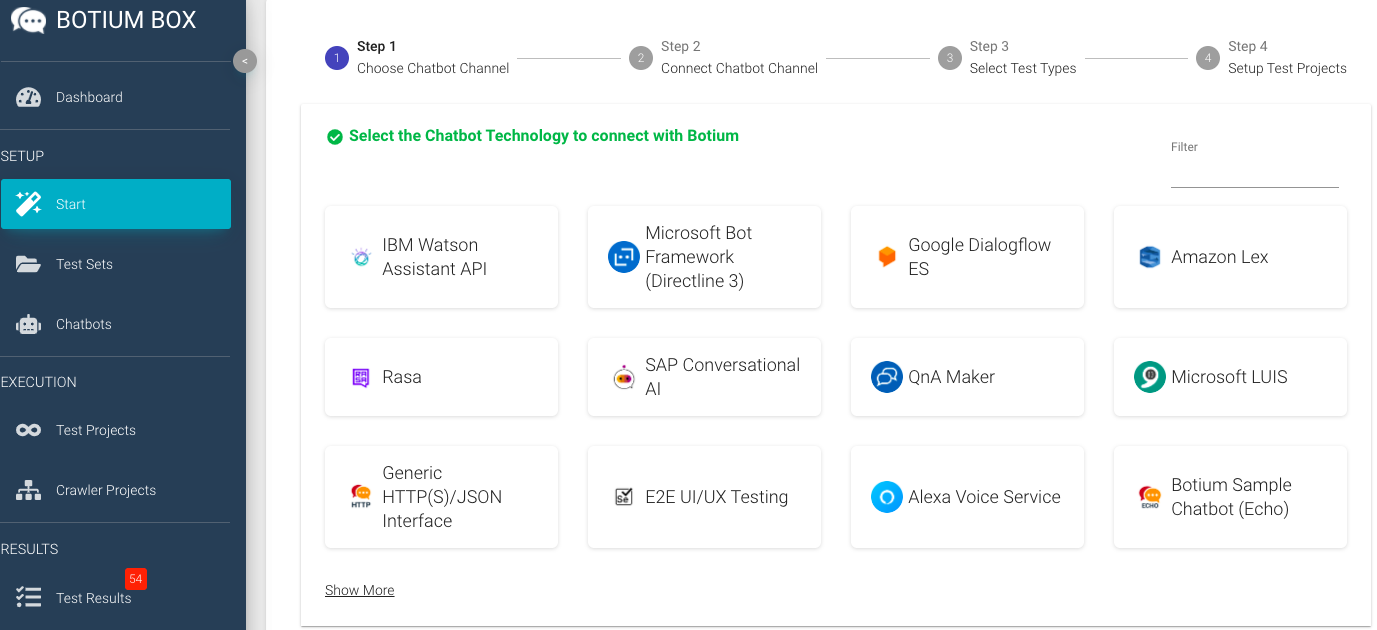
Botium.ai is a trailblazing service that confers you with the power to comprehensively test your chatbot. It furnishes you with a sophisticated simulator that facilitates input of messages, thereby enabling you to scrutinize and scrutinize the manner in which your chatbot responds. This cutting-edge feature effectively allows you to conduct an all-encompassing chatbot testing procedure, thereby guaranteeing the expected levels of performance upon chatbot deployment.
FAQs on Chatbot Development
Is chatbot using Python better than using other programming languages for developing chatbots?
Determining whether Python reigns supreme over other programming languages when it comes to crafting chatbots is a veritable Pandora’s box. The answer hinges on a multitude of factors such as the requirements at hand and individual preferences.
What are some of the most popular Python libraries for developing chatbots?
When it comes to developing chatbots using Python, the plethora of options at your disposal can be overwhelming. Nonetheless, several libraries have risen to the top echelon of popularity. Notable among these are NLTK, ChatterBot, and Botkit.
What are some of the most popular chatbot platforms?
Once your chatbot is ready for deployment, a wide array of platforms are available to you. Facebook Messenger, Telegram, Slack, and Kik are some of the most prominent and widely utilized chatbot platforms. The final choice ultimately depends on the specifics of your use case and desired outcomes.
How much does it cost to develop a chatbot?
When it comes to assessing the cost of developing a chatbot, several factors come into play that affect the price tag. Well, firstly, the important thing to consider is the complexity of the chatbot and the platform that the chatbot is being deployed on. If you are to ask us, it would roughly cost anywhere between $500 to $5000, give or take.
How long does it take to develop a chatbot using python?
Chatbots using Python, as simple as it may seem, the amount of time required can be quite difficult to accurate. The timeline of the bot development can vary greatly depending on the complexity of the chatbot. You can say that you might spend it might take from a week to a couple of months, depending on the architecture of the bot.
Are there any risks associated with developing chatbots?
Developing chatbots isn’t without its risks, either. Privacy and security risks, such as nefarious actors using chatbots to collect sensitive or personal data, are present. To minimize these hazards, implementing appropriate privacy and security measures is crucial.
Is there a future for chatbots?
The future looks exceedingly bright for chatbots, as they gain momentum and popularity across a multitude of industries, including but not limited to customer service, sales, and marketing. Furthermore, chatbots are poised to become even more advanced as Artificial Intelligence and Natural Language Processing technology continues to progress, leading to a more sophisticated and nuanced chatbot experience that can understand and respond to customer needs with greater accuracy and efficiency.
Conclusion
This discourse has explored the process of developing and deploying a Python-based chatbot, along with the potential benefits of using chatbots in various industries. Once your chatbot is fully functional, it can be utilized for a wide range of purposes, from providing customer support to facilitating sales and marketing efforts.
If you find yourself uncertain of where to begin in your quest for a customized chatbot, consider the advantageous option of enlisting the aid of a chatbot development agency. Such a move would undoubtedly provide you with the necessary guidance to create a chatbot that satisfies your specific needs. However, it’s crucial to conduct thorough research to locate a reputable agency that can meet your expectations.
At 12 Channels, we take pride in our expertise in creating bespoke chatbots for your enterprise. Our team of seasoned chatbot developers can expertly guide you through the complex and multifaceted process of creating a chatbot that precisely fits your unique objectives. Our unwavering dedication to delivering high-quality services and exceeding our clients’ expectations has earned us a reputation as a reliable and trustworthy chatbot development agency.
If you’re looking to delve deeper into the benefits of utilizing our services, please do not hesitate to contact us today. Thank you for taking the time to read this article, and we hope you have fun with the intricate art of coding!